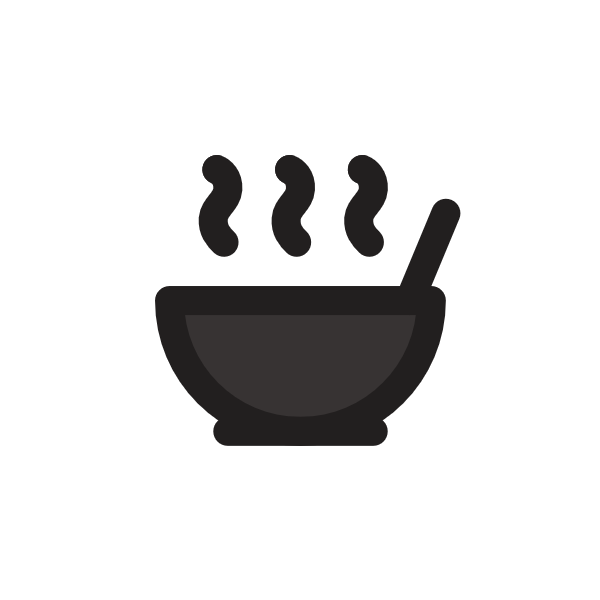
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Spaghetti code
Read the original article here.
The Forbidden Code: Unraveling the Spaghetti Monster
Welcome, initiates, to a dive into the murky depths of code that school won't teach you how to write, but will expect you to understand (and often, fix). Forget clean architectures and perfectly layered abstractions for a moment. Today, we confront the Spaghetti Monster of programming: Spaghetti Code.
While not a "forbidden technique" you'd intentionally master, understanding spaghetti code is crucial. It's the legacy you might inherit, the nightmare project you might land on, or the trap you could fall into if you're not careful. Learning to recognize it, understand its origins, and, most importantly, escape its clutches, is a vital, underground skill.
What is Spaghetti Code? The Tangled Mess Defined
Spaghetti Code: A pejorative term used to describe source code that is unstructured, poorly organized, and difficult to understand or maintain. Its complexity often arises from convoluted control flow paths, excessive jumping between distant parts of the code, and a general lack of clear logical separation. The name evokes the image of a bowl of spaghetti – tangled, with no clear beginning or end to any single strand.
Think of the clean, linear paths you're shown in introductory programming: functions calling functions, loops iterating through data, conditional statements branching logically. Spaghetti code throws all that out the window. It's code where the execution jumps around seemingly arbitrarily, making it almost impossible to trace the program's logic flow from start to finish.
The Primary Culprit: The GOTO Statement (and its Modern Relatives)
Historically, the most notorious driver of spaghetti code was the GOTO
statement.
GOTO Statement: A control flow statement found in many programming languages that causes an unconditional jump to a specified label or line number within the program.
In the early days of programming, before sophisticated structured control flow statements (like if-then-else
, for
loops, while
loops, and proper function calls/returns) were commonplace or widely adopted, GOTO
was often the primary tool for controlling program execution. Need to skip some lines? GOTO
a label further down. Need to repeat a block? GOTO
a label further up.
While GOTO
can technically implement any control flow, its unrestricted nature allows for jumps into and out of any point in the code. This makes it incredibly difficult to reason about the program's state or execution path at any given point. You lose the clear entry and exit points that structured constructs provide.
Example: Classic GOTO-driven Spaghetti (BASIC)
Consider this simple task: print numbers 1 to 10 and their squares.
10 I = 1
20 PRINT I, I*I
30 I = I + 1
40 IF I <= 10 THEN GOTO 20
50 END
This might seem simple, but even here, the logic flows via jumping (GOTO 20
). In a real-world program with hundreds or thousands of lines, multiple GOTO
s pointing in different directions create an impenetrable web.
The Structured Alternative:
FOR I = 1 TO 10
PRINT I, I*I
NEXT I
END
This version uses a FOR
loop, a structured construct. The loop has a clear entry point (the FOR
line), a clear condition for continuation (TO 10
), an automatic increment (NEXT I
), and a clear exit point (after NEXT I
once the condition is met). The flow is contained and predictable within the loop construct itself.
Modern Spaghetti (Beyond GOTO):
While direct GOTO
usage has significantly declined in mainstream languages (many don't even have it), the spirit of spaghetti code persists. It can manifest as:
- Excessively long functions or methods: Code blocks that try to do too much, becoming a tangled mess of logic.
- Deeply nested conditional statements:
if/else if/else
blocks nested many levels deep, creating complex branches. - Arbitrary flags and global variables: Logic that depends on tracking numerous flags or modifying global state from various points in the code, making it hard to follow dependencies.
- Misuse of exceptions for control flow: Using
try/catch
blocks not for handling errors, but for jumping out of expected execution paths. - Object-Oriented Spaghetti: Creating classes that are essentially just containers for long, procedural methods, ignoring concepts like encapsulation, inheritance, and polymorphism.
The Historical Context: Why Did Spaghetti Code Happen?
Understanding the history helps us see why this "forbidden" style emerged.
- Early Programming Limitations: In the very early days, programming involved writing machine code or assembly. To fix an error or insert instructions, programmers often couldn't just "insert" lines – they'd have to shift all subsequent code and update every single address that referred to the moved instructions. The workaround was to use a jump (
GOTO
) to an unused memory location, put the new/corrected code there, and then jump back. Repeat this a few times, and the execution path literally looked like tangled spaghetti on a memory map. Richard Hamming's description captures this perfectly. - Lack of High-Level Constructs: Early languages and programming paradigms simply didn't offer the rich set of structured control flow options we have today (
while
,for
, clear function call stacks).GOTO
was the primitive tool available. - The "Code and Fix" Model: Before formal software development methodologies like the Waterfall model or Agile, development was often a less planned "code and fix" process. You'd write some code, test it, find bugs, patch them, find more bugs, patch them... This iterative patching often led to quick, localized fixes using
GOTO
or other immediate means, accumulating technical debt and tangled code over time. - Volatile Requirements: Projects with constantly changing requirements, especially without disciplined refactoring, encourage quick, often messy patches to add new features or alter existing logic, leading to code entropy and spaghetti.
- Developer Skill and Experience: Inexperienced programmers may not be aware of or understand the benefits of structured programming principles. Lack of team-wide programming style rules also contributes to inconsistency and messiness.
The structured programming movement, notably championed by Edsger Dijkstra (whose famous paper "Go To Statement Considered Harmful" is a landmark), arose precisely as a reaction against the chaos and difficulty of managing GOTO
-heavy spaghetti code. Languages like Pascal and Ada were designed with features that enforced more structured approaches.
Related Pasta: Other Code Anti-Patterns
Spaghetti code is the most famous "pasta" anti-pattern, but you might encounter others in the wild:
Ravioli Code: Specific to object-oriented programming. Describes a system composed of many small, well-encapsulated classes (like individual ravioli pieces) that are easy to understand in isolation, but whose interactions and overall system flow are complex and hard to grasp. The system's behavior isn't obvious from looking at individual classes.
Lasagna Code: Describes code with too many layers of abstraction, where making a simple change requires navigating and modifying code across multiple layers. It's like digging through many thin layers of pasta, sauce, and cheese to reach the bottom. Changes aren't localized within a layer but ripple throughout the system.
These terms highlight that while GOTO
is the classic spaghetti maker, poor structure can manifest in many ways, even within paradigms like OOP.
The Cost of Spaghetti Code: Why It's "Forbidden" to Be Efficient
While no one actively tries to write spaghetti code (usually), its consequences make it something to actively avoid. In the "Forbidden Code" real world, this is where spaghetti code really bites you:
- Maintenance Nightmares: This is the biggest cost. Fixing bugs or adding new features becomes incredibly difficult and time-consuming. Tracing the execution path to understand why something is happening is a detective job requiring immense patience. A simple change in one place can have unpredictable side effects elsewhere due to the tangled dependencies.
- Increased Bug Density: The complexity and lack of clarity make it easy to introduce new bugs when trying to modify the code. The hidden dependencies mean fixing one issue can easily create another.
- Slow Development Velocity: New features take longer to implement because developers struggle to understand the existing codebase and ensure their changes don't break anything.
- Difficulty in Onboarding: New team members take much longer to become productive because the barrier to understanding the codebase is so high.
- Technical Debt Accumulation: Spaghetti code is a prime example of technical debt. The initial "save time now" approach (using
GOTO
, quick patches) leads to exponentially higher costs later.
Conclusion: Escaping the Spaghetti Trap
Spaghetti code isn't an "underground technique" you learn to use, but rather a phenomenon you must understand to combat. It's the chaotic result of insufficient discipline, historical constraints, or pressure to deliver quickly without considering long-term maintainability.
While schools teach structured programming, which seems "clean" and perhaps overly academic, its true value becomes brutally apparent when you encounter a real-world spaghetti codebase. Structured programming, with its clear control flow, modularity, and separation of concerns, is your best weapon against the Spaghetti Monster.
Recognizing spaghetti code, understanding how it got there, and knowing the principles of structured programming and refactoring are essential survival skills in the programming jungle. Don't get tangled up – strive for code you (and others) can follow, maintain, and build upon. That's the truly powerful, un-forbidden knowledge.
Related Articles
See Also
- "Amazon codewhisperer chat history missing"
- "Amazon codewhisperer keeps freezing mid-response"
- "Amazon codewhisperer keeps logging me out"
- "Amazon codewhisperer not generating code properly"
- "Amazon codewhisperer not loading past responses"
- "Amazon codewhisperer not responding"
- "Amazon codewhisperer not writing full answers"
- "Amazon codewhisperer outputs blank response"
- "Amazon codewhisperer vs amazon codewhisperer comparison"
- "Are ai apps safe"